- All Implemented Interfaces:
Styleable
,EventTarget
,Skinnable
@DefaultProperty("pages") public class Pagination extends Control
A Pagination control is used for navigation between pages of a single content, which has been divided into smaller parts.
Styling the page indicators
The control can be customized to display numeric page indicators or bullet style indicators by
setting the style class STYLE_CLASS_BULLET
. The
maxPageIndicatorCountProperty
can be used to change
the maximum number of page indicators. The property value can also be changed
via CSS using -fx-max-page-indicator-count
. By default, page indicator numbering starts from 1 (corresponding to
page index 0).
Page count
The pageCountProperty
controls the number of
pages this pagination control has. If the page count is
not known, INDETERMINATE
should be used as the page count.
Page factory
The pageFactoryProperty
is a callback function
that is called when a page has been selected by the application or
the user. The function is required for the functionality of the pagination
control. The callback function should load and return the contents of the selected page.
null
should be returned if the selected page index does not exist.
Creating a Pagination control:
A simple example of how to create a pagination control with ten pages and each page containing text.
Pagination pagination = new Pagination(10, 0);
pagination.setPageFactory(new Callback<Integer, Node>() {
@Override
public Node call(Integer pageIndex) {
return new Label(pageIndex + 1 + ". Lorem ipsum dolor sit amet,\n"
+ "consectetur adipiscing elit,\n"
+ "sed do eiusmod tempor incididunt ut\n"
+ "labore et dolore magna aliqua.");
}
});
or using lambdas
Pagination pagination = new Pagination(10, 0);
pagination.setPageFactory(pageIndex ->
new Label(pageIndex + 1 + ". Lorem ipsum dolor sit amet,\n"
+ "consectetur adipiscing elit,\n"
+ "sed do eiusmod tempor incididunt ut\n"
+ "labore et dolore magna aliqua.");
);
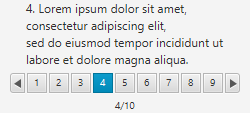
- Since:
- JavaFX 2.2
-
Property Summary
Properties Type Property Description IntegerProperty
currentPageIndex
The current page index to display for this pagination control.IntegerProperty
maxPageIndicatorCount
The maximum number of page indicators to use for this pagination control.IntegerProperty
pageCount
The number of pages for this pagination control.ObjectProperty<Callback<Integer,Node>>
pageFactory
The pageFactory callback function that is called when a page has been selected by the application or the user.Properties inherited from class javafx.scene.control.Control
contextMenu, skin, tooltip
Properties inherited from class javafx.scene.layout.Region
background, border, cacheShape, centerShape, height, insets, maxHeight, maxWidth, minHeight, minWidth, opaqueInsets, padding, prefHeight, prefWidth, scaleShape, shape, snapToPixel, width
Properties inherited from class javafx.scene.Parent
needsLayout
Properties inherited from class javafx.scene.Node
accessibleHelp, accessibleRoleDescription, accessibleRole, accessibleText, blendMode, boundsInLocal, boundsInParent, cacheHint, cache, clip, cursor, depthTest, disabled, disable, effectiveNodeOrientation, effect, eventDispatcher, focused, focusTraversable, hover, id, inputMethodRequests, layoutBounds, layoutX, layoutY, localToParentTransform, localToSceneTransform, managed, mouseTransparent, nodeOrientation, onContextMenuRequested, onDragDetected, onDragDone, onDragDropped, onDragEntered, onDragExited, onDragOver, onInputMethodTextChanged, onKeyPressed, onKeyReleased, onKeyTyped, onMouseClicked, onMouseDragEntered, onMouseDragExited, onMouseDragged, onMouseDragOver, onMouseDragReleased, onMouseEntered, onMouseExited, onMouseMoved, onMousePressed, onMouseReleased, onRotate, onRotationFinished, onRotationStarted, onScrollFinished, onScroll, onScrollStarted, onSwipeDown, onSwipeLeft, onSwipeRight, onSwipeUp, onTouchMoved, onTouchPressed, onTouchReleased, onTouchStationary, onZoomFinished, onZoom, onZoomStarted, opacity, parent, pickOnBounds, pressed, rotate, rotationAxis, scaleX, scaleY, scaleZ, scene, style, translateX, translateY, translateZ, viewOrder, visible
-
Field Summary
Fields Modifier and Type Field Description static int
INDETERMINATE
Value for indicating that the page count is indeterminate.static String
STYLE_CLASS_BULLET
The style class to change the numeric page indicators to bullet indicators.Fields inherited from class javafx.scene.layout.Region
USE_COMPUTED_SIZE, USE_PREF_SIZE
Fields inherited from class javafx.scene.Node
BASELINE_OFFSET_SAME_AS_HEIGHT
-
Constructor Summary
Constructors Constructor Description Pagination()
Constructs a Pagination control with anINDETERMINATE
page count and a page index equal to zero.Pagination(int pageCount)
Constructs a new Pagination control with the specified page count.Pagination(int pageCount, int pageIndex)
Constructs a new Pagination control with the specified page count and page index. -
Method Summary
Modifier and Type Method Description protected Skin<?>
createDefaultSkin()
Create a new instance of the default skin for this control.IntegerProperty
currentPageIndexProperty()
The current page index to display for this pagination control.static List<CssMetaData<? extends Styleable,?>>
getClassCssMetaData()
List<CssMetaData<? extends Styleable,?>>
getControlCssMetaData()
int
getCurrentPageIndex()
Returns the current page index.int
getMaxPageIndicatorCount()
Returns the maximum number of page indicators.int
getPageCount()
Returns the number of pages.Callback<Integer,Node>
getPageFactory()
Returns the page factory callback function.IntegerProperty
maxPageIndicatorCountProperty()
The maximum number of page indicators to use for this pagination control.IntegerProperty
pageCountProperty()
The number of pages for this pagination control.ObjectProperty<Callback<Integer,Node>>
pageFactoryProperty()
The pageFactory callback function that is called when a page has been selected by the application or the user.void
setCurrentPageIndex(int value)
Sets the current page index.void
setMaxPageIndicatorCount(int value)
Sets the maximum number of page indicators.void
setPageCount(int value)
Sets the number of pages.void
setPageFactory(Callback<Integer,Node> value)
Sets the page factory callback function.Methods inherited from class javafx.scene.control.Control
computeMaxHeight, computeMaxWidth, computeMinHeight, computeMinWidth, computePrefHeight, computePrefWidth, contextMenuProperty, executeAccessibleAction, getBaselineOffset, getContextMenu, getCssMetaData, getInitialFocusTraversable, getSkin, getTooltip, isResizable, layoutChildren, queryAccessibleAttribute, setContextMenu, setSkin, setTooltip, skinProperty, tooltipProperty
Methods inherited from class javafx.scene.layout.Region
backgroundProperty, borderProperty, cacheShapeProperty, centerShapeProperty, getBackground, getBorder, getHeight, getInsets, getMaxHeight, getMaxWidth, getMinHeight, getMinWidth, getOpaqueInsets, getPadding, getPrefHeight, getPrefWidth, getShape, getUserAgentStylesheet, getWidth, heightProperty, insetsProperty, isCacheShape, isCenterShape, isScaleShape, isSnapToPixel, layoutInArea, layoutInArea, layoutInArea, layoutInArea, maxHeight, maxHeightProperty, maxWidth, maxWidthProperty, minHeight, minHeightProperty, minWidth, minWidthProperty, opaqueInsetsProperty, paddingProperty, positionInArea, positionInArea, prefHeight, prefHeightProperty, prefWidth, prefWidthProperty, resize, scaleShapeProperty, setBackground, setBorder, setCacheShape, setCenterShape, setHeight, setMaxHeight, setMaxSize, setMaxWidth, setMinHeight, setMinSize, setMinWidth, setOpaqueInsets, setPadding, setPrefHeight, setPrefSize, setPrefWidth, setScaleShape, setShape, setSnapToPixel, setWidth, shapeProperty, snappedBottomInset, snappedLeftInset, snappedRightInset, snappedTopInset, snapPosition, snapPositionX, snapPositionY, snapSize, snapSizeX, snapSizeY, snapSpace, snapSpaceX, snapSpaceY, snapToPixelProperty, widthProperty
Methods inherited from class javafx.scene.Parent
getChildren, getChildrenUnmodifiable, getManagedChildren, getStylesheets, isNeedsLayout, layout, lookup, needsLayoutProperty, requestLayout, requestParentLayout, setNeedsLayout, updateBounds
Methods inherited from class javafx.scene.Node
accessibleHelpProperty, accessibleRoleDescriptionProperty, accessibleRoleProperty, accessibleTextProperty, addEventFilter, addEventHandler, applyCss, autosize, blendModeProperty, boundsInLocalProperty, boundsInParentProperty, buildEventDispatchChain, cacheHintProperty, cacheProperty, clipProperty, computeAreaInScreen, contains, contains, cursorProperty, depthTestProperty, disabledProperty, disableProperty, effectiveNodeOrientationProperty, effectProperty, eventDispatcherProperty, fireEvent, focusedProperty, focusTraversableProperty, getAccessibleHelp, getAccessibleRole, getAccessibleRoleDescription, getAccessibleText, getBlendMode, getBoundsInLocal, getBoundsInParent, getCacheHint, getClip, getContentBias, getCursor, getDepthTest, getEffect, getEffectiveNodeOrientation, getEventDispatcher, getId, getInitialCursor, getInputMethodRequests, getLayoutBounds, getLayoutX, getLayoutY, getLocalToParentTransform, getLocalToSceneTransform, getNodeOrientation, getOnContextMenuRequested, getOnDragDetected, getOnDragDone, getOnDragDropped, getOnDragEntered, getOnDragExited, getOnDragOver, getOnInputMethodTextChanged, getOnKeyPressed, getOnKeyReleased, getOnKeyTyped, getOnMouseClicked, getOnMouseDragEntered, getOnMouseDragExited, getOnMouseDragged, getOnMouseDragOver, getOnMouseDragReleased, getOnMouseEntered, getOnMouseExited, getOnMouseMoved, getOnMousePressed, getOnMouseReleased, getOnRotate, getOnRotationFinished, getOnRotationStarted, getOnScroll, getOnScrollFinished, getOnScrollStarted, getOnSwipeDown, getOnSwipeLeft, getOnSwipeRight, getOnSwipeUp, getOnTouchMoved, getOnTouchPressed, getOnTouchReleased, getOnTouchStationary, getOnZoom, getOnZoomFinished, getOnZoomStarted, getOpacity, getParent, getProperties, getPseudoClassStates, getRotate, getRotationAxis, getScaleX, getScaleY, getScaleZ, getScene, getStyle, getStyleableParent, getStyleClass, getTransforms, getTranslateX, getTranslateY, getTranslateZ, getTypeSelector, getUserData, getViewOrder, hasProperties, hoverProperty, idProperty, inputMethodRequestsProperty, intersects, intersects, isCache, isDisable, isDisabled, isFocused, isFocusTraversable, isHover, isManaged, isMouseTransparent, isPickOnBounds, isPressed, isVisible, layoutBoundsProperty, layoutXProperty, layoutYProperty, localToParent, localToParent, localToParent, localToParent, localToParent, localToParentTransformProperty, localToScene, localToScene, localToScene, localToScene, localToScene, localToScene, localToScene, localToScene, localToScene, localToScene, localToSceneTransformProperty, localToScreen, localToScreen, localToScreen, localToScreen, localToScreen, lookupAll, managedProperty, mouseTransparentProperty, nodeOrientationProperty, notifyAccessibleAttributeChanged, onContextMenuRequestedProperty, onDragDetectedProperty, onDragDoneProperty, onDragDroppedProperty, onDragEnteredProperty, onDragExitedProperty, onDragOverProperty, onInputMethodTextChangedProperty, onKeyPressedProperty, onKeyReleasedProperty, onKeyTypedProperty, onMouseClickedProperty, onMouseDragEnteredProperty, onMouseDragExitedProperty, onMouseDraggedProperty, onMouseDragOverProperty, onMouseDragReleasedProperty, onMouseEnteredProperty, onMouseExitedProperty, onMouseMovedProperty, onMousePressedProperty, onMouseReleasedProperty, onRotateProperty, onRotationFinishedProperty, onRotationStartedProperty, onScrollFinishedProperty, onScrollProperty, onScrollStartedProperty, onSwipeDownProperty, onSwipeLeftProperty, onSwipeRightProperty, onSwipeUpProperty, onTouchMovedProperty, onTouchPressedProperty, onTouchReleasedProperty, onTouchStationaryProperty, onZoomFinishedProperty, onZoomProperty, onZoomStartedProperty, opacityProperty, parentProperty, parentToLocal, parentToLocal, parentToLocal, parentToLocal, parentToLocal, pickOnBoundsProperty, pressedProperty, pseudoClassStateChanged, relocate, removeEventFilter, removeEventHandler, requestFocus, resizeRelocate, rotateProperty, rotationAxisProperty, scaleXProperty, scaleYProperty, scaleZProperty, sceneProperty, sceneToLocal, sceneToLocal, sceneToLocal, sceneToLocal, sceneToLocal, sceneToLocal, sceneToLocal, sceneToLocal, screenToLocal, screenToLocal, screenToLocal, setAccessibleHelp, setAccessibleRole, setAccessibleRoleDescription, setAccessibleText, setBlendMode, setCache, setCacheHint, setClip, setCursor, setDepthTest, setDisable, setDisabled, setEffect, setEventDispatcher, setEventHandler, setFocused, setFocusTraversable, setHover, setId, setInputMethodRequests, setLayoutX, setLayoutY, setManaged, setMouseTransparent, setNodeOrientation, setOnContextMenuRequested, setOnDragDetected, setOnDragDone, setOnDragDropped, setOnDragEntered, setOnDragExited, setOnDragOver, setOnInputMethodTextChanged, setOnKeyPressed, setOnKeyReleased, setOnKeyTyped, setOnMouseClicked, setOnMouseDragEntered, setOnMouseDragExited, setOnMouseDragged, setOnMouseDragOver, setOnMouseDragReleased, setOnMouseEntered, setOnMouseExited, setOnMouseMoved, setOnMousePressed, setOnMouseReleased, setOnRotate, setOnRotationFinished, setOnRotationStarted, setOnScroll, setOnScrollFinished, setOnScrollStarted, setOnSwipeDown, setOnSwipeLeft, setOnSwipeRight, setOnSwipeUp, setOnTouchMoved, setOnTouchPressed, setOnTouchReleased, setOnTouchStationary, setOnZoom, setOnZoomFinished, setOnZoomStarted, setOpacity, setPickOnBounds, setPressed, setRotate, setRotationAxis, setScaleX, setScaleY, setScaleZ, setStyle, setTranslateX, setTranslateY, setTranslateZ, setUserData, setViewOrder, setVisible, snapshot, snapshot, startDragAndDrop, startFullDrag, styleProperty, toBack, toFront, toString, translateXProperty, translateYProperty, translateZProperty, usesMirroring, viewOrderProperty, visibleProperty
Methods inherited from class java.lang.Object
clone, equals, finalize, getClass, hashCode, notify, notifyAll, wait, wait, wait
Methods inherited from interface javafx.css.Styleable
getStyleableNode
-
Property Details
-
maxPageIndicatorCount
The maximum number of page indicators to use for this pagination control. The maximum number of pages indicators will remain unchanged if the value is less than 1 or greater than thepageCount
. The number of page indicators will be reduced to fit the control if themaxPageIndicatorCount
cannot fit. The default is 10 page indicators. -
pageCount
The number of pages for this pagination control. This value must be greater than or equal to 1.INDETERMINATE
should be used as the page count if the total number of pages is unknown. The default is anINDETERMINATE
number of pages.- See Also:
getPageCount()
,setPageCount(int)
-
currentPageIndex
The current page index to display for this pagination control. The first page will be the current page if the value is less than 0. Similarly the last page will be the current page if the value is greater than thepageCount
The default is 0 for the first page.Because the page indicators set the current page index, the currentPageIndex property permits only bidirectional binding. The
bind
method throws an UnsupportedOperationException.- See Also:
getCurrentPageIndex()
,setCurrentPageIndex(int)
-
pageFactory
The pageFactory callback function that is called when a page has been selected by the application or the user. This function is required for the functionality of the pagination control. The callback function should load and return the contents the page index. Null should be returned if the page index does not exist. The currentPageIndex will not change when null is returned. The default is null if there is no page factory set.- See Also:
getPageFactory()
,setPageFactory(Callback)
-
-
Field Details
-
STYLE_CLASS_BULLET
The style class to change the numeric page indicators to bullet indicators.- See Also:
- Constant Field Values
-
INDETERMINATE
public static final int INDETERMINATEValue for indicating that the page count is indeterminate.- See Also:
setPageCount(int)
, Constant Field Values
-
-
Constructor Details
-
Pagination
public Pagination(int pageCount, int pageIndex)Constructs a new Pagination control with the specified page count and page index.- Parameters:
pageCount
- the number of pages for the pagination controlpageIndex
- the index of the first page.
-
Pagination
public Pagination(int pageCount)Constructs a new Pagination control with the specified page count.- Parameters:
pageCount
- the number of pages for the pagination control
-
Pagination
public Pagination()Constructs a Pagination control with anINDETERMINATE
page count and a page index equal to zero.
-
-
Method Details
-
setMaxPageIndicatorCount
public final void setMaxPageIndicatorCount(int value)Sets the maximum number of page indicators.- Parameters:
value
- the number of page indicators. The default is 10.
-
getMaxPageIndicatorCount
public final int getMaxPageIndicatorCount()Returns the maximum number of page indicators.- Returns:
- the maximum number of page indicators
-
maxPageIndicatorCountProperty
The maximum number of page indicators to use for this pagination control. The maximum number of pages indicators will remain unchanged if the value is less than 1 or greater than thepageCount
. The number of page indicators will be reduced to fit the control if themaxPageIndicatorCount
cannot fit. The default is 10 page indicators. -
setPageCount
public final void setPageCount(int value)Sets the number of pages.- Parameters:
value
- the number of pages
-
getPageCount
public final int getPageCount()Returns the number of pages.- Returns:
- the number of pages
-
pageCountProperty
The number of pages for this pagination control. This value must be greater than or equal to 1.INDETERMINATE
should be used as the page count if the total number of pages is unknown. The default is anINDETERMINATE
number of pages.- See Also:
getPageCount()
,setPageCount(int)
-
setCurrentPageIndex
public final void setCurrentPageIndex(int value)Sets the current page index.- Parameters:
value
- the current page index.
-
getCurrentPageIndex
public final int getCurrentPageIndex()Returns the current page index.- Returns:
- the current page index
-
currentPageIndexProperty
The current page index to display for this pagination control. The first page will be the current page if the value is less than 0. Similarly the last page will be the current page if the value is greater than thepageCount
The default is 0 for the first page.Because the page indicators set the current page index, the currentPageIndex property permits only bidirectional binding. The
bind
method throws an UnsupportedOperationException.- See Also:
getCurrentPageIndex()
,setCurrentPageIndex(int)
-
setPageFactory
Sets the page factory callback function.- Parameters:
value
- the page factory callback function
-
getPageFactory
Returns the page factory callback function.- Returns:
- the page factory callback function
-
pageFactoryProperty
The pageFactory callback function that is called when a page has been selected by the application or the user. This function is required for the functionality of the pagination control. The callback function should load and return the contents the page index. Null should be returned if the page index does not exist. The currentPageIndex will not change when null is returned. The default is null if there is no page factory set.- See Also:
getPageFactory()
,setPageFactory(Callback)
-
createDefaultSkin
Create a new instance of the default skin for this control. This is called to create a skin for the control if no skin is provided via CSS-fx-skin
or set explicitly in a sub-class withsetSkin(...)
.- Overrides:
createDefaultSkin
in classControl
- Returns:
- new instance of default skin for this control. If null then the control will have no skin unless one is provided by css.
-
getClassCssMetaData
- Returns:
- The CssMetaData associated with this class, which may include the CssMetaData of its superclasses.
- Since:
- JavaFX 8.0
-
getControlCssMetaData
- Overrides:
getControlCssMetaData
in classControl
- Returns:
- unmodifiable list of the controls css styleable properties
- Since:
- JavaFX 8.0
-