- All Implemented Interfaces:
Styleable
,EventTarget
,Toggle
public class RadioMenuItem extends MenuItem implements Toggle
A RadioMenuItem is a MenuItem
that can be toggled (it uses
the Toggle
mixin). This means that
RadioMenuItem has an API very similar in nature to other controls that use
Toggle
, such as
RadioButton
and
ToggleButton
. RadioMenuItem is
specifically designed for use within a Menu
, so refer to that class
API documentation for more information on how to add a RadioMenuItem into it.
To create a simple, ungrouped RadioMenuItem, do the following:
RadioMenuItem radioItem = new RadioMenuItem("radio text");
radioItem.setSelected(false);
radioItem.setOnAction(e -> System.out.println("radio toggled"));
The problem with the example above is that this offers no benefit over using
a normal MenuItem. As already mentioned, the purpose of a
RadioMenuItem is to offer
multiple choices to the user, and only allow for one of these choices to be
selected at any one time (i.e. the selection should be mutually exclusive).
To achieve this, you can place zero or more RadioMenuItem's into groups. When
in groups, only one RadioMenuItem at a time within that group can be selected.
To put two RadioMenuItem instances into the same group, simply assign them
both the same value for toggleGroup
. For example:
ToggleGroup toggleGroup = new ToggleGroup();
RadioMenuItem radioItem1 = new RadioMenuItem("Option 1");
radioItem1.setOnAction(e -> System.out.println("radio1 toggled"));
radioItem1.setToggleGroup(toggleGroup);
RadioMenuItem radioItem2 = new RadioMenuItem("Option 2");
radioItem2.setOnAction(e -> System.out.println("radio2 toggled"));
radioItem2.setToggleGroup(toggleGroup);
Menu menu = new Menu("Selection");
menu.getItems().addAll(radioItem1, radioItem2);
MenuBar menuBar = new MenuBar(menu);
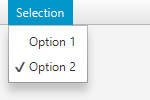
In this example, with both RadioMenuItem's assigned to the same
ToggleGroup
, only one item may be
selected at any one time, and should
the selection change, the ToggleGroup will take care of deselecting the
previous item.
-
Property Summary
Properties Type Property Description BooleanProperty
selected
The selected state for thisToggle
.ObjectProperty<ToggleGroup>
toggleGroup
Represents theToggleGroup
that this RadioMenuItem belongs to.Properties inherited from class javafx.scene.control.MenuItem
accelerator, disable, graphic, id, mnemonicParsing, onAction, onMenuValidation, parentMenu, parentPopup, style, text, visible
-
Field Summary
Fields inherited from class javafx.scene.control.MenuItem
MENU_VALIDATION_EVENT
-
Constructor Summary
Constructors Constructor Description RadioMenuItem()
Constructs a RadioMenuItem with no display text.RadioMenuItem(String text)
Constructs a RadioMenuItem and sets the display text with the specified text.RadioMenuItem(String text, Node graphic)
Constructs a RadioMenuItem and sets the display text with the specified text and sets the graphicNode
to the given node. -
Method Summary
Modifier and Type Method Description ToggleGroup
getToggleGroup()
Gets the value of the property toggleGroup.boolean
isSelected()
Gets the value of the property selected.BooleanProperty
selectedProperty()
The selected state for thisToggle
.void
setSelected(boolean value)
Sets the value of the property selected.void
setToggleGroup(ToggleGroup value)
Sets the value of the property toggleGroup.ObjectProperty<ToggleGroup>
toggleGroupProperty()
Represents theToggleGroup
that this RadioMenuItem belongs to.Methods inherited from class javafx.scene.control.MenuItem
acceleratorProperty, addEventHandler, buildEventDispatchChain, disableProperty, fire, getAccelerator, getCssMetaData, getGraphic, getId, getOnAction, getOnMenuValidation, getParentMenu, getParentPopup, getProperties, getPseudoClassStates, getStyle, getStyleableNode, getStyleableParent, getStyleClass, getText, getTypeSelector, getUserData, graphicProperty, idProperty, isDisable, isMnemonicParsing, isVisible, mnemonicParsingProperty, onActionProperty, onMenuValidationProperty, parentMenuProperty, parentPopupProperty, removeEventHandler, setAccelerator, setDisable, setGraphic, setId, setMnemonicParsing, setOnAction, setOnMenuValidation, setParentMenu, setParentPopup, setStyle, setText, setUserData, setVisible, styleProperty, textProperty, toString, visibleProperty
Methods inherited from class java.lang.Object
clone, equals, finalize, getClass, hashCode, notify, notifyAll, wait, wait, wait
Methods inherited from interface javafx.scene.control.Toggle
getProperties, getUserData, setUserData
-
Property Details
-
toggleGroup
Represents theToggleGroup
that this RadioMenuItem belongs to.- Specified by:
toggleGroupProperty
in interfaceToggle
- See Also:
getToggleGroup()
,setToggleGroup(ToggleGroup)
-
selected
- Specified by:
selectedProperty
in interfaceToggle
- See Also:
isSelected()
,setSelected(boolean)
-
-
Constructor Details
-
RadioMenuItem
public RadioMenuItem()Constructs a RadioMenuItem with no display text. -
RadioMenuItem
Constructs a RadioMenuItem and sets the display text with the specified text.- Parameters:
text
- the display text
-
RadioMenuItem
Constructs a RadioMenuItem and sets the display text with the specified text and sets the graphicNode
to the given node.- Parameters:
text
- the display textgraphic
- the graphic node
-
-
Method Details
-
setToggleGroup
Sets the value of the property toggleGroup.- Specified by:
setToggleGroup
in interfaceToggle
- Property description:
- Represents the
ToggleGroup
that this RadioMenuItem belongs to. - Parameters:
value
- The newToggleGroup
.
-
getToggleGroup
Gets the value of the property toggleGroup.- Specified by:
getToggleGroup
in interfaceToggle
- Property description:
- Represents the
ToggleGroup
that this RadioMenuItem belongs to. - Returns:
- The
ToggleGroup
to which thisToggle
belongs.
-
toggleGroupProperty
Represents theToggleGroup
that this RadioMenuItem belongs to.- Specified by:
toggleGroupProperty
in interfaceToggle
- See Also:
getToggleGroup()
,setToggleGroup(ToggleGroup)
-
setSelected
public final void setSelected(boolean value)Sets the value of the property selected.- Specified by:
setSelected
in interfaceToggle
- Property description:
- Parameters:
value
-true
to make thisToggle
selected.
-
isSelected
public final boolean isSelected()Gets the value of the property selected.- Specified by:
isSelected
in interfaceToggle
- Property description:
- Returns:
true
if thisToggle
is selected.
-
selectedProperty
Description copied from interface:Toggle
The selected state for thisToggle
.- Specified by:
selectedProperty
in interfaceToggle
- See Also:
isSelected()
,setSelected(boolean)
-