- All Implemented Interfaces:
Styleable
,EventTarget
- Direct Known Subclasses:
CheckMenuItem
,CustomMenuItem
,Menu
,RadioMenuItem
@IDProperty("id") public class MenuItem extends Object implements EventTarget, Styleable
MenuItem is intended to be used in conjunction with Menu
to provide
options to users. MenuItem serves as the base class for the bulk of JavaFX menus
API.
It has a display text
property, as well as an optional graphic
node
that can be set on it.
The accelerator
property enables accessing the
associated action in one keystroke. Also, as with the Button
control,
by using the setOnAction(javafx.event.EventHandler<javafx.event.ActionEvent>)
method, you can have an instance of MenuItem
perform any action you wish.
Note: Whilst any size of graphic can be inserted into a MenuItem, the most commonly used size in most applications is 16x16 pixels. This is the recommended graphic dimension to use if you're using the default style provided by JavaFX.
To create a MenuItem is simple:
MenuItem menuItem = new MenuItem("Open");
menuItem.setOnAction(e -> System.out.println("Opening Database Connection..."));
Circle graphic = new Circle(8);
graphic.setFill(Color.GREEN);
menuItem.setGraphic(graphic);
Menu menu = new Menu("File");
menu.getItems().add(menuItem);
MenuBar menuBar = new MenuBar(menu);
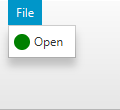
- Since:
- JavaFX 2.0
- See Also:
Menu
-
Property Summary
Properties Type Property Description ObjectProperty<KeyCombination>
accelerator
The accelerator property enables accessing the associated action in one keystroke.BooleanProperty
disable
Sets the individual disabled state of this MenuItem.ObjectProperty<Node>
graphic
An optional graphic for theMenuItem
.StringProperty
id
The id of this MenuItem.BooleanProperty
mnemonicParsing
MnemonicParsing property to enable/disable text parsing.ObjectProperty<EventHandler<ActionEvent>>
onAction
The action, which is invoked whenever the MenuItem is fired.ObjectProperty<EventHandler<Event>>
onMenuValidation
The event handler that is associated with invocation of an accelerator for a MenuItem.ReadOnlyObjectProperty<Menu>
parentMenu
This is theMenu
in which thisMenuItem
exists.ReadOnlyObjectProperty<ContextMenu>
parentPopup
This is theContextMenu
in which thisMenuItem
exists.StringProperty
style
A string representation of the CSS style associated with this specific MenuItem.StringProperty
text
The text to display in theMenuItem
.BooleanProperty
visible
Specifies whether this MenuItem should be rendered as part of the scene graph. -
Field Summary
Fields Modifier and Type Field Description static EventType<Event>
MENU_VALIDATION_EVENT
Called when a accelerator for the Menuitem is invoked -
Constructor Summary
Constructors Constructor Description MenuItem()
Constructs a MenuItem with no display text.MenuItem(String text)
Constructs a MenuItem and sets the display text with the specified textMenuItem(String text, Node graphic)
Constructor s MenuItem and sets the display text with the specified text and sets the graphicNode
to the given node. -
Method Summary
Modifier and Type Method Description ObjectProperty<KeyCombination>
acceleratorProperty()
The accelerator property enables accessing the associated action in one keystroke.<E extends Event>
voidaddEventHandler(EventType<E> eventType, EventHandler<E> eventHandler)
Registers an event handler to this MenuItem.EventDispatchChain
buildEventDispatchChain(EventDispatchChain tail)
Construct an event dispatch chain for this target.BooleanProperty
disableProperty()
Sets the individual disabled state of this MenuItem.void
fire()
Fires a new ActionEvent.KeyCombination
getAccelerator()
Gets the value of the property accelerator.List<CssMetaData<? extends Styleable,?>>
getCssMetaData()
The CssMetaData of this Styleable.Node
getGraphic()
Gets the value of the property graphic.String
getId()
Gets the value of the property id.EventHandler<ActionEvent>
getOnAction()
Gets the value of the property onAction.EventHandler<Event>
getOnMenuValidation()
Gets the value of the property onMenuValidation.Menu
getParentMenu()
Gets the value of the property parentMenu.ContextMenu
getParentPopup()
Gets the value of the property parentPopup.ObservableMap<Object,Object>
getProperties()
Returns an observable map of properties on this menu item for use primarily by application developers.ObservableSet<PseudoClass>
getPseudoClassStates()
Return the pseudo-class state of this Styleable.String
getStyle()
Gets the value of the property style.Node
getStyleableNode()
Returns the Node that represents this Styleable object.Styleable
getStyleableParent()
Return the parent of this Styleable, or null if there is no parent.ObservableList<String>
getStyleClass()
* Public API * *String
getText()
Gets the value of the property text.String
getTypeSelector()
The type of thisStyleable
that is to be used in selector matching.Object
getUserData()
ObjectProperty<Node>
graphicProperty()
An optional graphic for theMenuItem
.StringProperty
idProperty()
The id of this MenuItem.boolean
isDisable()
Gets the value of the property disable.boolean
isMnemonicParsing()
Gets the value of the property mnemonicParsing.boolean
isVisible()
Gets the value of the property visible.BooleanProperty
mnemonicParsingProperty()
MnemonicParsing property to enable/disable text parsing.ObjectProperty<EventHandler<ActionEvent>>
onActionProperty()
The action, which is invoked whenever the MenuItem is fired.ObjectProperty<EventHandler<Event>>
onMenuValidationProperty()
The event handler that is associated with invocation of an accelerator for a MenuItem.ReadOnlyObjectProperty<Menu>
parentMenuProperty()
This is theMenu
in which thisMenuItem
exists.ReadOnlyObjectProperty<ContextMenu>
parentPopupProperty()
This is theContextMenu
in which thisMenuItem
exists.<E extends Event>
voidremoveEventHandler(EventType<E> eventType, EventHandler<E> eventHandler)
Unregisters a previously registered event handler from this MenuItem.void
setAccelerator(KeyCombination value)
Sets the value of the property accelerator.void
setDisable(boolean value)
Sets the value of the property disable.void
setGraphic(Node value)
Sets the value of the property graphic.void
setId(String value)
Sets the value of the property id.void
setMnemonicParsing(boolean value)
Sets the value of the property mnemonicParsing.void
setOnAction(EventHandler<ActionEvent> value)
Sets the value of the property onAction.void
setOnMenuValidation(EventHandler<Event> value)
Sets the value of the property onMenuValidation.protected void
setParentMenu(Menu value)
Sets the value of the property parentMenu.protected void
setParentPopup(ContextMenu value)
Sets the value of the property parentPopup.void
setStyle(String value)
Sets the value of the property style.void
setText(String value)
Sets the value of the property text.void
setUserData(Object value)
Stores a user object that contains data at their discretion.void
setVisible(boolean value)
Sets the value of the property visible.StringProperty
styleProperty()
A string representation of the CSS style associated with this specific MenuItem.StringProperty
textProperty()
The text to display in theMenuItem
.String
toString()
BooleanProperty
visibleProperty()
Specifies whether this MenuItem should be rendered as part of the scene graph.
-
Property Details
-
id
The id of this MenuItem. This simple string identifier is useful for finding a specific MenuItem within the scene graph.- See Also:
getId()
,setId(String)
-
style
A string representation of the CSS style associated with this specific MenuItem. This is analogous to the "style" attribute of an HTML element. Note that, like the HTML style attribute, this variable contains style properties and values and not the selector portion of a style rule.- See Also:
getStyle()
,setStyle(String)
-
parentMenu
This is theMenu
in which thisMenuItem
exists. It is possible for an instance of this class to not have aparentMenu
- this means that this instance is either:- Not yet associated with its
parentMenu
. - A 'root'
Menu
(i.e. it is a context menu, attached directly to aMenuBar
,MenuButton
, or any of the other controls that useMenu
internally.
- See Also:
getParentMenu()
,setParentMenu(Menu)
- Not yet associated with its
-
parentPopup
This is theContextMenu
in which thisMenuItem
exists.- See Also:
getParentPopup()
,setParentPopup(ContextMenu)
-
text
The text to display in theMenuItem
.- See Also:
getText()
,setText(String)
-
graphic
An optional graphic for theMenuItem
. This will normally be anImageView
node, but there is no requirement for this to be the case.- See Also:
getGraphic()
,setGraphic(Node)
-
onAction
The action, which is invoked whenever the MenuItem is fired. This may be due to the user clicking on the button with the mouse, or by a touch event, or by a key press, or if the developer programatically invokes thefire()
method.- See Also:
getOnAction()
,setOnAction(EventHandler)
-
onMenuValidation
The event handler that is associated with invocation of an accelerator for a MenuItem. This can happen when a key sequence for an accelerator is pressed. The event handler is also invoked when onShowing event handler is called.- Since:
- JavaFX 2.2
- See Also:
getOnMenuValidation()
,setOnMenuValidation(EventHandler)
-
disable
Sets the individual disabled state of this MenuItem. Setting disable to true will cause this MenuItem to become disabled.- See Also:
isDisable()
,setDisable(boolean)
-
visible
Specifies whether this MenuItem should be rendered as part of the scene graph.- See Also:
isVisible()
,setVisible(boolean)
-
accelerator
The accelerator property enables accessing the associated action in one keystroke. It is a convenience offered to perform quickly a given action.- See Also:
getAccelerator()
,setAccelerator(KeyCombination)
-
mnemonicParsing
MnemonicParsing property to enable/disable text parsing. If this is set to true, then the MenuItem text will be parsed to see if it contains the mnemonic parsing character '_'. When a mnemonic is detected the key combination will be determined based on the succeeding character, and the mnemonic added.The default value for MenuItem is true.
- See Also:
isMnemonicParsing()
,setMnemonicParsing(boolean)
-
-
Field Details
-
MENU_VALIDATION_EVENT
Called when a accelerator for the Menuitem is invoked
- Since:
- JavaFX 2.2
-
-
Constructor Details
-
MenuItem
public MenuItem()Constructs a MenuItem with no display text. -
MenuItem
Constructs a MenuItem and sets the display text with the specified text- Parameters:
text
- the display text- See Also:
setText(java.lang.String)
-
MenuItem
Constructor s MenuItem and sets the display text with the specified text and sets the graphicNode
to the given node.- Parameters:
text
- the display textgraphic
- the graphic node- See Also:
setText(java.lang.String)
,setGraphic(javafx.scene.Node)
-
-
Method Details
-
setId
Sets the value of the property id.- Property description:
- The id of this MenuItem. This simple string identifier is useful for finding a specific MenuItem within the scene graph.
-
getId
Gets the value of the property id. -
idProperty
The id of this MenuItem. This simple string identifier is useful for finding a specific MenuItem within the scene graph.- See Also:
getId()
,setId(String)
-
setStyle
Sets the value of the property style.- Property description:
- A string representation of the CSS style associated with this specific MenuItem. This is analogous to the "style" attribute of an HTML element. Note that, like the HTML style attribute, this variable contains style properties and values and not the selector portion of a style rule.
-
getStyle
Gets the value of the property style.- Specified by:
getStyle
in interfaceStyleable
- Property description:
- A string representation of the CSS style associated with this specific MenuItem. This is analogous to the "style" attribute of an HTML element. Note that, like the HTML style attribute, this variable contains style properties and values and not the selector portion of a style rule.
- Returns:
- a string representation of the CSS style associated with this
specific
Node
-
styleProperty
A string representation of the CSS style associated with this specific MenuItem. This is analogous to the "style" attribute of an HTML element. Note that, like the HTML style attribute, this variable contains style properties and values and not the selector portion of a style rule.- See Also:
getStyle()
,setStyle(String)
-
setParentMenu
Sets the value of the property parentMenu.- Property description:
- This is the
Menu
in which thisMenuItem
exists. It is possible for an instance of this class to not have aparentMenu
- this means that this instance is either:- Not yet associated with its
parentMenu
. - A 'root'
Menu
(i.e. it is a context menu, attached directly to aMenuBar
,MenuButton
, or any of the other controls that useMenu
internally.
- Not yet associated with its
-
getParentMenu
Gets the value of the property parentMenu.- Property description:
- This is the
Menu
in which thisMenuItem
exists. It is possible for an instance of this class to not have aparentMenu
- this means that this instance is either:- Not yet associated with its
parentMenu
. - A 'root'
Menu
(i.e. it is a context menu, attached directly to aMenuBar
,MenuButton
, or any of the other controls that useMenu
internally.
- Not yet associated with its
-
parentMenuProperty
This is theMenu
in which thisMenuItem
exists. It is possible for an instance of this class to not have aparentMenu
- this means that this instance is either:- Not yet associated with its
parentMenu
. - A 'root'
Menu
(i.e. it is a context menu, attached directly to aMenuBar
,MenuButton
, or any of the other controls that useMenu
internally.
- See Also:
getParentMenu()
,setParentMenu(Menu)
- Not yet associated with its
-
setParentPopup
Sets the value of the property parentPopup.- Property description:
- This is the
ContextMenu
in which thisMenuItem
exists.
-
getParentPopup
Gets the value of the property parentPopup.- Property description:
- This is the
ContextMenu
in which thisMenuItem
exists.
-
parentPopupProperty
This is theContextMenu
in which thisMenuItem
exists.- See Also:
getParentPopup()
,setParentPopup(ContextMenu)
-
setText
Sets the value of the property text.- Property description:
- The text to display in the
MenuItem
.
-
getText
Gets the value of the property text.- Property description:
- The text to display in the
MenuItem
.
-
textProperty
The text to display in theMenuItem
.- See Also:
getText()
,setText(String)
-
setGraphic
Sets the value of the property graphic.- Property description:
- An optional graphic for the
MenuItem
. This will normally be anImageView
node, but there is no requirement for this to be the case.
-
getGraphic
Gets the value of the property graphic.- Property description:
- An optional graphic for the
MenuItem
. This will normally be anImageView
node, but there is no requirement for this to be the case.
-
graphicProperty
An optional graphic for theMenuItem
. This will normally be anImageView
node, but there is no requirement for this to be the case.- See Also:
getGraphic()
,setGraphic(Node)
-
setOnAction
Sets the value of the property onAction.- Property description:
- The action, which is invoked whenever the MenuItem is fired. This
may be due to the user clicking on the button with the mouse, or by
a touch event, or by a key press, or if the developer programatically
invokes the
fire()
method.
-
getOnAction
Gets the value of the property onAction.- Property description:
- The action, which is invoked whenever the MenuItem is fired. This
may be due to the user clicking on the button with the mouse, or by
a touch event, or by a key press, or if the developer programatically
invokes the
fire()
method.
-
onActionProperty
The action, which is invoked whenever the MenuItem is fired. This may be due to the user clicking on the button with the mouse, or by a touch event, or by a key press, or if the developer programatically invokes thefire()
method.- See Also:
getOnAction()
,setOnAction(EventHandler)
-
setOnMenuValidation
Sets the value of the property onMenuValidation.- Property description:
- The event handler that is associated with invocation of an accelerator for a MenuItem. This can happen when a key sequence for an accelerator is pressed. The event handler is also invoked when onShowing event handler is called.
- Since:
- JavaFX 2.2
-
getOnMenuValidation
Gets the value of the property onMenuValidation.- Property description:
- The event handler that is associated with invocation of an accelerator for a MenuItem. This can happen when a key sequence for an accelerator is pressed. The event handler is also invoked when onShowing event handler is called.
- Since:
- JavaFX 2.2
-
onMenuValidationProperty
The event handler that is associated with invocation of an accelerator for a MenuItem. This can happen when a key sequence for an accelerator is pressed. The event handler is also invoked when onShowing event handler is called.- Since:
- JavaFX 2.2
- See Also:
getOnMenuValidation()
,setOnMenuValidation(EventHandler)
-
setDisable
public final void setDisable(boolean value)Sets the value of the property disable.- Property description:
- Sets the individual disabled state of this MenuItem. Setting disable to true will cause this MenuItem to become disabled.
-
isDisable
public final boolean isDisable()Gets the value of the property disable.- Property description:
- Sets the individual disabled state of this MenuItem. Setting disable to true will cause this MenuItem to become disabled.
-
disableProperty
Sets the individual disabled state of this MenuItem. Setting disable to true will cause this MenuItem to become disabled.- See Also:
isDisable()
,setDisable(boolean)
-
setVisible
public final void setVisible(boolean value)Sets the value of the property visible.- Property description:
- Specifies whether this MenuItem should be rendered as part of the scene graph.
-
isVisible
public final boolean isVisible()Gets the value of the property visible.- Property description:
- Specifies whether this MenuItem should be rendered as part of the scene graph.
-
visibleProperty
Specifies whether this MenuItem should be rendered as part of the scene graph.- See Also:
isVisible()
,setVisible(boolean)
-
setAccelerator
Sets the value of the property accelerator.- Property description:
- The accelerator property enables accessing the associated action in one keystroke. It is a convenience offered to perform quickly a given action.
-
getAccelerator
Gets the value of the property accelerator.- Property description:
- The accelerator property enables accessing the associated action in one keystroke. It is a convenience offered to perform quickly a given action.
-
acceleratorProperty
The accelerator property enables accessing the associated action in one keystroke. It is a convenience offered to perform quickly a given action.- See Also:
getAccelerator()
,setAccelerator(KeyCombination)
-
setMnemonicParsing
public final void setMnemonicParsing(boolean value)Sets the value of the property mnemonicParsing.- Property description:
- MnemonicParsing property to enable/disable text parsing.
If this is set to true, then the MenuItem text will be
parsed to see if it contains the mnemonic parsing character '_'.
When a mnemonic is detected the key combination will
be determined based on the succeeding character, and the mnemonic
added.
The default value for MenuItem is true.
-
isMnemonicParsing
public final boolean isMnemonicParsing()Gets the value of the property mnemonicParsing.- Property description:
- MnemonicParsing property to enable/disable text parsing.
If this is set to true, then the MenuItem text will be
parsed to see if it contains the mnemonic parsing character '_'.
When a mnemonic is detected the key combination will
be determined based on the succeeding character, and the mnemonic
added.
The default value for MenuItem is true.
-
mnemonicParsingProperty
MnemonicParsing property to enable/disable text parsing. If this is set to true, then the MenuItem text will be parsed to see if it contains the mnemonic parsing character '_'. When a mnemonic is detected the key combination will be determined based on the succeeding character, and the mnemonic added.The default value for MenuItem is true.
- See Also:
isMnemonicParsing()
,setMnemonicParsing(boolean)
-
getStyleClass
* Public API * *- Specified by:
getStyleClass
in interfaceStyleable
- Returns:
- a list of String identifiers which can be used to logically group Nodes, specifically for an external style engine
- See Also:
- CSS3 class selectors
-
fire
public void fire()Fires a new ActionEvent. -
addEventHandler
public <E extends Event> void addEventHandler(EventType<E> eventType, EventHandler<E> eventHandler)Registers an event handler to this MenuItem. The handler is called when the menu item receives anEvent
of the specified type during the bubbling phase of event delivery.- Type Parameters:
E
- the specific event class of the handler- Parameters:
eventType
- the type of the events to receive by the handlereventHandler
- the handler to register- Throws:
NullPointerException
- if the event type or handler is null
-
removeEventHandler
public <E extends Event> void removeEventHandler(EventType<E> eventType, EventHandler<E> eventHandler)Unregisters a previously registered event handler from this MenuItem. One handler might have been registered for different event types, so the caller needs to specify the particular event type from which to unregister the handler.- Type Parameters:
E
- the specific event class of the handler- Parameters:
eventType
- the event type from which to unregistereventHandler
- the handler to unregister- Throws:
NullPointerException
- if the event type or handler is null
-
buildEventDispatchChain
Construct an event dispatch chain for this target. The event dispatch chain contains event dispatchers which might be interested in processing of events targeted at thisEventTarget
. This event target is not automatically added to the chain, so if it wants to process events, it needs to add anEventDispatcher
for itself to the chain.In the case the event target is part of some hierarchy, the chain for it is usually built from event dispatchers collected from the root of the hierarchy to the event target.
The event dispatch chain is constructed by modifications to the provided initial event dispatch chain. The returned chain should have the initial chain at its end so the dispatchers should be prepended to the initial chain.
The caller shouldn't assume that the initial chain remains unchanged nor that the returned value will reference a different chain.
- Specified by:
buildEventDispatchChain
in interfaceEventTarget
- Parameters:
tail
- the initial chain to build from- Returns:
- the resulting event dispatch chain for this target
-
getUserData
- Returns:
- the user object that was stored (including
null
), ornull
if no object has been set
-
setUserData
Stores a user object that contains data at their discretion. It can later be retrieved by callinggetUserData()
.- Parameters:
value
- the user object to be stored
-
getProperties
Returns an observable map of properties on this menu item for use primarily by application developers.- Returns:
- an observable map of properties on this menu item for use primarily by application developers
-
getTypeSelector
The type of thisStyleable
that is to be used in selector matching. This is analogous to an "element" in HTML. (CSS Type Selector).- Specified by:
getTypeSelector
in interfaceStyleable
- Returns:
- "MenuItem"
- Since:
- JavaFX 8.0
-
getStyleableParent
Return the parent of this Styleable, or null if there is no parent.- Specified by:
getStyleableParent
in interfaceStyleable
- Returns:
getParentMenu()
, orgetParentPopup()
ifparentMenu
is null- Since:
- JavaFX 8.0
-
getPseudoClassStates
Return the pseudo-class state of this Styleable. CSS assumes this set is read-only.- Specified by:
getPseudoClassStates
in interfaceStyleable
- Returns:
- the pseudo-class state
- Since:
- JavaFX 8.0
-
getCssMetaData
Description copied from interface:Styleable
The CssMetaData of this Styleable. This may be returned as an unmodifiable list.- Specified by:
getCssMetaData
in interfaceStyleable
- Returns:
- the CssMetaData
-
getStyleableNode
Returns the Node that represents this Styleable object. This method should be overridden in cases where the Styleable is not itself a Node, so that it may optionally return the relevant root node representation of itself. By default this method returns null, which can mean that either the Styleable itself is a Node, or if that is not the case, that the Styleable does not have a node representation available at the time of request.- Specified by:
getStyleableNode
in interfaceStyleable
- Returns:
- the Node that represents this Styleable object
-
toString
-