- All Implemented Interfaces:
Styleable
,EventTarget
,Skinnable
@IDProperty("id") public class ContextMenu extends PopupControl
A popup control containing an ObservableList of menu items. The items
ObservableList allows for any MenuItem
type to be inserted,
including its subclasses Menu
, MenuItem
, RadioMenuItem
, CheckMenuItem
and
CustomMenuItem
. If an arbitrary Node needs to be
inserted into a menu, a CustomMenuItem can be used. One exception to this general rule is that
SeparatorMenuItem
could be used for inserting a separator.
A common use case for this class is creating and showing context menus to users. To create a context menu using ContextMenu you can do the following:
final ContextMenu contextMenu = new ContextMenu();
contextMenu.setOnShowing(new EventHandler<WindowEvent>() {
public void handle(WindowEvent e) {
System.out.println("showing");
}
});
contextMenu.setOnShown(new EventHandler<WindowEvent>() {
public void handle(WindowEvent e) {
System.out.println("shown");
}
});
MenuItem item1 = new MenuItem("About");
item1.setOnAction(new EventHandler<ActionEvent>() {
public void handle(ActionEvent e) {
System.out.println("About");
}
});
MenuItem item2 = new MenuItem("Preferences");
item2.setOnAction(new EventHandler<ActionEvent>() {
public void handle(ActionEvent e) {
System.out.println("Preferences");
}
});
contextMenu.getItems().addAll(item1, item2);
final TextField textField = new TextField("Type Something");
textField.setContextMenu(contextMenu);
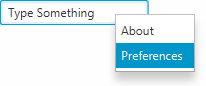
Control.setContextMenu(javafx.scene.control.ContextMenu)
convenience
method can be used to set a context menu on on any control. The example above results in the
context menu being displayed on the right Side
of the TextField. Alternatively, an event handler can also be set on the control
to invoke the context menu as shown below.
textField.setOnAction(new EventHandler<ActionEvent>() {
public void handle(ActionEvent e) {
contextMenu.show(textField, Side.BOTTOM, 0, 0);
}
});
Group root = (Group) scene.getRoot();
root.getChildren().add(textField);
In this example, the context menu is shown when the user clicks on the
Button
(of course, you should use the
MenuButton
control to do this rather than doing the above).
Note that the show function used in the code sample
above will result in the ContextMenu appearing directly beneath the
TextField. You can vary the Side
to get the results you expect.
-
Property Summary
Properties Type Property Description ObjectProperty<EventHandler<ActionEvent>>
onAction
Callback function to be informed when an item contained within thisContextMenu
has been activated.Properties inherited from class javafx.scene.control.PopupControl
id, maxHeight, maxWidth, minHeight, minWidth, prefHeight, prefWidth, skin, style
Properties inherited from class javafx.stage.PopupWindow
anchorLocation, anchorX, anchorY, autoFix, autoHide, consumeAutoHidingEvents, hideOnEscape, onAutoHide, ownerNode, ownerWindow
Properties inherited from class javafx.stage.Window
eventDispatcher, focused, forceIntegerRenderScale, height, onCloseRequest, onHidden, onHiding, onShowing, onShown, opacity, outputScaleX, outputScaleY, renderScaleX, renderScaleY, scene, showing, width, x, y
-
Nested Class Summary
Nested classes/interfaces inherited from class javafx.scene.control.PopupControl
PopupControl.CSSBridge
Nested classes/interfaces inherited from class javafx.stage.PopupWindow
PopupWindow.AnchorLocation
-
Field Summary
Fields inherited from class javafx.scene.control.PopupControl
bridge, USE_COMPUTED_SIZE, USE_PREF_SIZE
-
Constructor Summary
Constructors Constructor Description ContextMenu()
Create a new ContextMenuContextMenu(MenuItem... items)
Create a new ContextMenu initialized with the given items -
Method Summary
Modifier and Type Method Description protected Skin<?>
createDefaultSkin()
Create a new instance of the default skin for this control.ObservableList<MenuItem>
getItems()
The menu items on the context menu.EventHandler<ActionEvent>
getOnAction()
Gets the value of the property onAction.void
hide()
Hides thisContextMenu
and any visible submenus, assuming that when this function is called that theContextMenu
was showing.ObjectProperty<EventHandler<ActionEvent>>
onActionProperty()
Callback function to be informed when an item contained within thisContextMenu
has been activated.void
setOnAction(EventHandler<ActionEvent> value)
Sets the value of the property onAction.void
show(Node anchor, double screenX, double screenY)
Shows theContextMenu
at the specified screen coordinates.void
show(Node anchor, Side side, double dx, double dy)
Shows theContextMenu
relative to the given anchor node, on the side specified by thehpos
andvpos
parameters, and offset by the givendx
anddy
values for the x-axis and y-axis, respectively.Methods inherited from class javafx.scene.control.PopupControl
getClassCssMetaData, getCssMetaData, getId, getMaxHeight, getMaxWidth, getMinHeight, getMinWidth, getPrefHeight, getPrefWidth, getPseudoClassStates, getSkin, getStyle, getStyleableNode, getStyleableParent, getStyleClass, getTypeSelector, idProperty, maxHeight, maxHeightProperty, maxWidth, maxWidthProperty, minHeight, minHeightProperty, minWidth, minWidthProperty, prefHeight, prefHeightProperty, prefWidth, prefWidthProperty, pseudoClassStateChanged, setId, setMaxHeight, setMaxSize, setMaxWidth, setMinHeight, setMinSize, setMinWidth, setPrefHeight, setPrefSize, setPrefWidth, setSkin, setStyle, skinProperty, styleProperty
Methods inherited from class javafx.stage.PopupWindow
anchorLocationProperty, anchorXProperty, anchorYProperty, autoFixProperty, autoHideProperty, consumeAutoHidingEventsProperty, getAnchorLocation, getAnchorX, getAnchorY, getConsumeAutoHidingEvents, getOnAutoHide, getOwnerNode, getOwnerWindow, hideOnEscapeProperty, isAutoFix, isAutoHide, isHideOnEscape, onAutoHideProperty, ownerNodeProperty, ownerWindowProperty, setAnchorLocation, setAnchorX, setAnchorY, setAutoFix, setAutoHide, setConsumeAutoHidingEvents, setHideOnEscape, setOnAutoHide, setScene, show, show
Methods inherited from class javafx.stage.Window
addEventFilter, addEventHandler, buildEventDispatchChain, centerOnScreen, eventDispatcherProperty, fireEvent, focusedProperty, forceIntegerRenderScaleProperty, getEventDispatcher, getHeight, getOnCloseRequest, getOnHidden, getOnHiding, getOnShowing, getOnShown, getOpacity, getOutputScaleX, getOutputScaleY, getProperties, getRenderScaleX, getRenderScaleY, getScene, getUserData, getWidth, getWindows, getX, getY, hasProperties, heightProperty, isFocused, isForceIntegerRenderScale, isShowing, onCloseRequestProperty, onHiddenProperty, onHidingProperty, onShowingProperty, onShownProperty, opacityProperty, outputScaleXProperty, outputScaleYProperty, removeEventFilter, removeEventHandler, renderScaleXProperty, renderScaleYProperty, requestFocus, sceneProperty, setEventDispatcher, setEventHandler, setForceIntegerRenderScale, setHeight, setOnCloseRequest, setOnHidden, setOnHiding, setOnShowing, setOnShown, setOpacity, setRenderScaleX, setRenderScaleY, setUserData, setWidth, setX, setY, show, showingProperty, sizeToScene, widthProperty, xProperty, yProperty
-
Property Details
-
onAction
Callback function to be informed when an item contained within thisContextMenu
has been activated. The current implementation informs all parent menus as well, so that it is not necessary to listen to all sub menus for events.- See Also:
getOnAction()
,setOnAction(EventHandler)
-
-
Constructor Details
-
ContextMenu
public ContextMenu()Create a new ContextMenu -
ContextMenu
Create a new ContextMenu initialized with the given items- Parameters:
items
- the list of menu items
-
-
Method Details
-
setOnAction
Sets the value of the property onAction.- Property description:
- Callback function to be informed when an item contained within this
ContextMenu
has been activated. The current implementation informs all parent menus as well, so that it is not necessary to listen to all sub menus for events.
-
getOnAction
Gets the value of the property onAction.- Property description:
- Callback function to be informed when an item contained within this
ContextMenu
has been activated. The current implementation informs all parent menus as well, so that it is not necessary to listen to all sub menus for events.
-
onActionProperty
Callback function to be informed when an item contained within thisContextMenu
has been activated. The current implementation informs all parent menus as well, so that it is not necessary to listen to all sub menus for events.- See Also:
getOnAction()
,setOnAction(EventHandler)
-
getItems
The menu items on the context menu. If this ObservableList is modified at runtime, the ContextMenu will update as expected.- Returns:
- the menu items on this context menu
- See Also:
MenuItem
-
show
Shows theContextMenu
relative to the given anchor node, on the side specified by thehpos
andvpos
parameters, and offset by the givendx
anddy
values for the x-axis and y-axis, respectively. If there is not enough room, the menu is moved to the opposite side and the offset is not applied.To clarify the purpose of the
hpos
andvpos
parameters, consider that they are relative to the anchor node. As such, ahpos
andvpos
ofCENTER
would mean that the ContextMenu appears on top of the anchor, with the (0,0) position of theContextMenu
positioned at (0,0) of the anchor. Ahpos
of right would then shift theContextMenu
such that its top-left (0,0) position would be attached to the top-right position of the anchor.This function is useful for finely tuning the position of a menu, relative to the parent node to ensure close alignment.
- Parameters:
anchor
- the anchor nodeside
- the sidedx
- the dx value for the x-axisdy
- the dy value for the y-axis
-
show
Shows theContextMenu
at the specified screen coordinates. If there is not enough room at the specified location to show theContextMenu
given its size requirements, the necessary adjustments are made to bring theContextMenu
back on screen. This also means that theContextMenu
will not span multiple monitors.- Overrides:
show
in classPopupWindow
- Parameters:
anchor
- the anchor nodescreenX
- the x position of the anchor in screen coordinatesscreenY
- the y position of the anchor in screen coordinates
-
hide
public void hide()Hides thisContextMenu
and any visible submenus, assuming that when this function is called that theContextMenu
was showing.If this
ContextMenu
is not showing, then nothing happens.- Overrides:
hide
in classPopupWindow
-
createDefaultSkin
Create a new instance of the default skin for this control. This is called to create a skin for the control if no skin is provided via CSS-fx-skin
or set explicitly in a sub-class withsetSkin(...)
.- Overrides:
createDefaultSkin
in classPopupControl
- Returns:
- new instance of default skin for this control. If null then the control will have no skin unless one is provided by css.
-